Predict Sentiment
Predicts sentiment in an input text.
Sentiment Analysis applies sentiment labels to text, which are returned at a sentence and document level, with a confidence score for each. The labels are positive, negative, and neutral. At the document level, the mixed sentiment label also can be returned. The sentiment of the document is determined below:
- At least one positive sentence is in the document. The rest of the sentences are neutral. positive
- At least one negative sentence is in the document. The rest of the sentences are neutral. negative
- At least one negative sentence and at least one positive sentence are in the document. mixed
- All sentences in the document are neutral. neutral
For this extraction we use Microsoft Azure Text Analytics api.
To be able to use this activity, we need an Api Key and an Endpoint URl.
You can use the existing keys from a Cognitive Services resource(if any available), or you can create a new one. To create a new resource, once you have the azure subscription, Create a Cognitive Services resource in the Azure portal to get your key and endpoint. After it deploys, click Go to resource. You will need the key and endpoint from the resource you create to connect your workflow to the Text Analytics API.
Below is a screenshot of Azure cognitive resource. Just copy one of the keys to Api Key and the EndPoint to Endpoint URl.
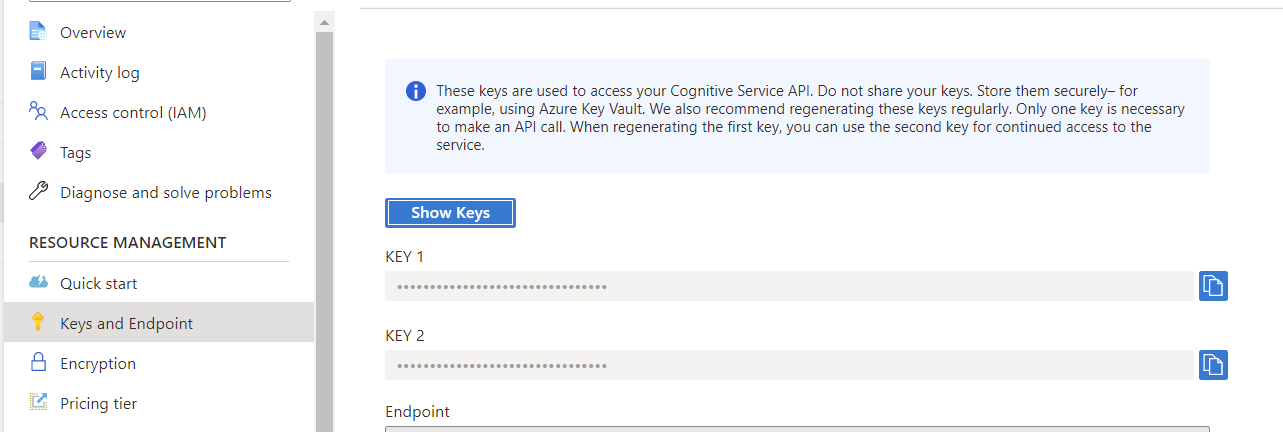
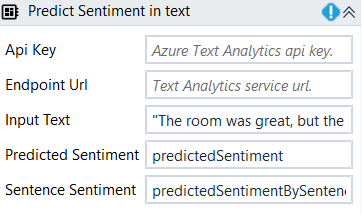
#
Designer PropertiesApi Key The Microsoft Azure api key from Cognitive Services resource. Please check above how to get this key.
Endpoint Url The Microsoft Azure Endpoint from Cognitive Services resource. Please check above how to get this key.
Input Text The text to be analysed.
Predicted Sentiment Predicted sentiment on the input text. It is represented with a Sentiment object with the following structure.
Sentence Sentiment Predicted sentiment by sentence. Represented as List(Sentiment).
#
Properties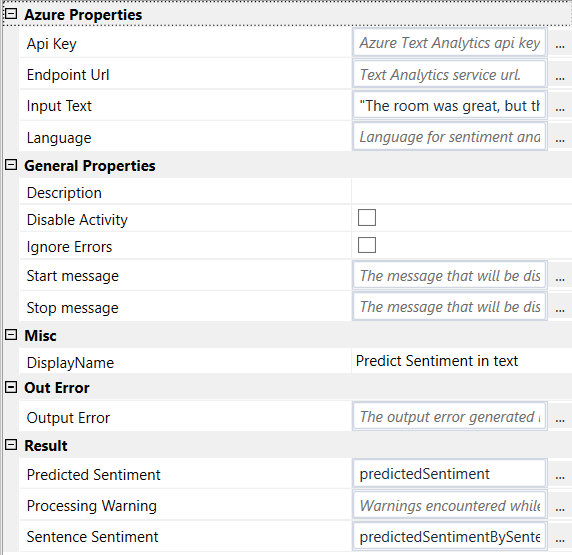
#
Azure Properties- See Designer Properties above.
- Language The language that the input text is written in. If unspecified, this value will be set to None. The list of supported languages can be found here https://docs.microsoft.com/en-us/azure/cognitive-services/text-analytics/language-support?tabs=sentiment-analysis Please use the Language code when setting this value.
#
General PropertiesSee General Properties.
#
MiscSee Misc.
#
Out ErrorSee Out Error.
#
Result- Predicted Sentiment See Designer Properties above.
- Processing Warnings The warnings encountered while processing the document represented as a List(SentimentWarning).
- Sentence Sentiment See Designer Properties above.
#
ExampleIn this example, we analyse the sentiment on the following phrase: "The room was great, but the staff was unfriendly" and display a message box with the result. At the time of testing, the sentiment returned was Negative with the value 0.97(from 1).
Please set the Api Key and Endpoint URl.