Web activities
Web activities group consists of activities specifically designed to interact with any web page. It includes activities, Send keys, mouse click or enumerate the items in a web page list or table. Web activities can recorded or dropped on the workflow from toolbox.
When recording web activities, we need to have either Chrome or Firefox Rinkt extension installed and enabled. The Rinkt Chrome extension is installed automatically, but it is not enabled(restriction from Google).
note
Most of the properties on these activities can be set using an expression. That is why when we set a string value, we must put that string value under "".
note
When searching the element at run time, we first try to use the Css path and if we cannot find the element using the Css path we fall back on the XPath. If we want to use only XPath, then we can just have to set the content of Css Path to empty.
Confirmation Dialog
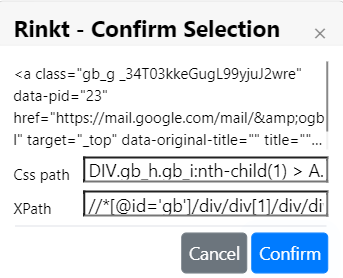
Most of the web activities share the following common properties:
#
Web Event Main Properties- Css Path The css path to find the element on the page. It is automatically generated when recording an element using an algorithm that calculates the score of every unique path and chooses the most optimum one, but for some elements we may have to change it manually. For pages that regenerate the ids, and some other element attributes on every visit, we may have to add some attributes that are the same on every visit.
- Ignore Url Ignores the url specified and looks for the element on the currently active browser page.
- Url The url of the page where to find the web element.
- Window Title The title of the web page that we want to access. It is used when trying to open a popup window from a main browser window in order to use the right window.
- XPath The XPath to find the element on the page. Like the css path, it is generated automatically using an algorithm similar with the one used by google Chrome debugger when recording, but in some cases we may have to edit it manually. For pages that regenerate the ids, and some other element attributes on every visit, we may have to add some attributes that are the same on every visit.
#
General Properties- Date and time of recording The date and time when the element was recorded (Readonly).
- Delay to start in ms The time to wait in milliseconds before starting to look for the element. This is used for some activities to wait for the element to become available.
- Description The activity description.
- Disable Activity Disables this activity. This means that no action will be performed for this activity. It is useful when implementing to disable some workflow activities and check the result.
- Element Index The index of the element to be selected. Some selectors may return multiple elements, and the index allows us to use a specific element.
- Ignore Errors Ignore errors if the current activity is failing and continues with the next activity. Even if the workflow continues to execute, we still report the activities in the log, console or error window.
- Number of Attempts Represents the number of attempts to find the element. If it is not set, we default to 3. After each retry, we wait retry index * 1 second(first retry 1 second, second retry 2 seconds ...).
- Screenshot file Name The name of the screenshot file taken at record time.
#
Out PropertiesAll of the following properties are set at workflow runtime with the details from the matched element.
- Attributes The element attributes at runtime, like id, class ..... It may be set to a variable of type ElementAttribute[] that may be created under Variables at the bottom of the workflow.
- Attributes Dictionary The element attributes at runtime, like id, class .... represented as Dictionary(string, string). It may be set to a variable of type Dictionary(string, string) that may be created under Variables at the bottom of the workflow.
- Children Tags The children tags for this element as a string[]. It may be set to a variable of type string[] that may be created under Variables at the bottom of the workflow.
- Client Height It is zero for elements with no CSS or inline layout boxes; otherwise, it's the inner height of an element in pixels. It includes padding but excludes borders, margins, and horizontal scrollbars (if present). It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Client Left The width of the left border of an element in pixels. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Client Top The width of the top border of the element in pixels. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Client Width It is zero for inline elements and elements with no CSS; otherwise, it's the inner width of an element in pixels. It includes padding but excludes borders, margins, and vertical scrollbars (if present). It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Inner HTML The HTML or XML markup contained within the element. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Inner Text The "rendered" text content of a node and its descendants. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Local Name The local part of the qualified name of this element. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- NameSpace URI The element name space URI. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Node Name The element node name. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Number of Children The number of children of this element. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Offset Height Typically, offsetHeight is a measurement in pixels of the element's CSS height, including any borders, padding, and horizontal scrollbars (if rendered). It does not include the height of pseudo-elements such as ::before or ::after. For the document body object, the measurement includes total linear content height instead of the element's CSS height. Floated elements extending below other linear content are ignored. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Offset Left The number of pixels that the upper left corner of the current element is offset to the left within the HTMLElement.offsetParent node. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Offset Top The distance of the outer border of the current element relative to the inner border of the top of the offsetParent node. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Offset Width Typically, offsetWidth is a measurement in pixels of the element's CSS width, including any borders, padding, and vertical scrollbars (if rendered). It does not include the width of pseudo-elements such as ::before or ::after. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Out Url The url for the page. It is useful when ignoring the Url and using the currently active browser page. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Outer HTML The serialized HTML fragment describing the element including its descendants. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Prefix The element namespace prefix. It may be set to a variable of type string that be may created under Variables at the bottom of the workflow.
- Scroll Left The number of pixels that an element's content is scrolled from its left edge. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Scroll Top The number of pixels that an element's content is scrolled vertically. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Scroll Width The width of the element's content, including content not visible on the screen due to overflow. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Scroll Height The height of an element's content, including content not visible on the screen due to overflow. It may be set to a variable of type Int32 that may be created under Variables at the bottom of the workflow.
- Tag Name The tag name of the element. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- Text The element text which includes the text from all children elements.
- Title The title of the element. It may be set to a variable of type string that may be created under Variables at the bottom of the workflow.
- value Element value when it is an input element.
- Was Successful Returns true if the element was found and false otherwise. If the value is set to a variable, the workflow will not be stopped when the element is not found. It may be set to a variable of type bool that may be created under Variables at the bottom of the workflow.
#
Web Event Advanced Properties- Css Full Path The css path from body to the element. It is populated automatically at recording, and it is used only if Use Css Full Path as Fallback is checked.
- Inner text The inner text of the element at recording.
- Use Css Full Path as Fallback Use Css Full path if the element cannot be found using Css Path or XPath.
- Use Debugger Send the event by simulating the human interaction with the element(Same as having a human clicking a button, but using the browser debugger).